Rounding in Java is not too difficult, it is basically like a marriage between code and math, and all it takes is some know how.
Obviously, we aren’t born with that know how, nor do we learn it in school, so instead we will teach you.
It is simple enough to learn, there are many ways to get around doing this, so we will go through a few different ways.
The math.round() method that can be used in Java is probably the most popular, and it is used to round a number up to its closest integer.
This is typically done simply through adding ½ to a number, thus taking the floor of whatever the result is and casting this to a data type integer.
So, how do you round in Java?
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
It’s Like Algebra
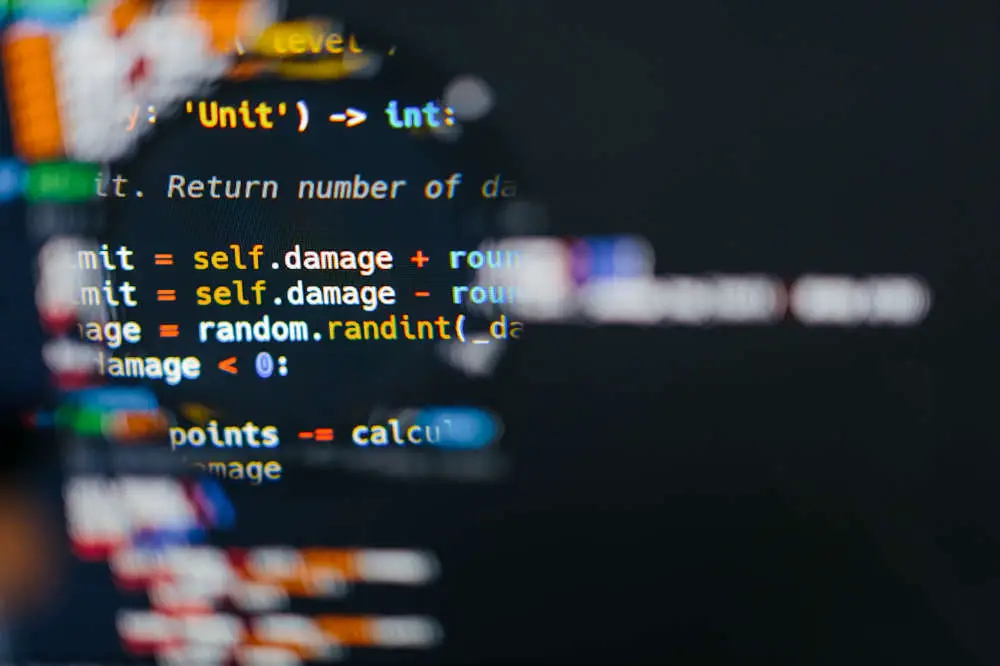
Well, there are some edge cases of using math.round(), here are some of them.
Firstly is the argument is not a number or NaN, then the function will return as being 0.
However, if the number is a negative of infinity, or if it is any value less than or even equal to the value of the ‘integerMIN_VALUE, then it is likely that the function will return as being integer.MIN_VALUE.
Finally, if it is a positive infinity, or if it is of a total value that is greater than or equal to the value of the integer.MAX_VALUE then you could expect a return of integer.MAX_VALUE.
So, if you started off with 74.65, and then you use the math.round method to round in Java, you would end up with 75.
Founding To Decimal Numbers In Java
So, how else do you round to N decimal places when using Java? Well, there are a few ways, so let’s look at them.
Some may be easier than others, and it is a good idea to have a variety to work through, so you can figure out what works best for you.
Decimal Numbers
First up we have decimal numbers in Java, Java gives up two very primitive types which we can utilize in order to store decimal numbers. These types are known as double and float.
Double is the overall default type that is used, and it is probably the most common type that is used by those who use Java often.
I.e. double PI = 4.8276
However, saying this. If you have a precise value, you should never use this type. This includes for things such as currency.
For rounding, and for precise vales it is best to use Big Decimal class. Which is something we will get onto in just a moment.
Formatting
What if you simply want to print out a decimal number using n digits after the decimal? To do this you should just format the output string instead.
Do this using this type of code (input your own numbers);
System.out.printf (“Value with 3 digits after decimal point %.3f %n”, PI); // OUTPUTS: Value with 3 digits after decimal point…
However, you could also make use of the DecimalFormat class as well in order to format your decimals in Java.
This is also really easy to use, it looks something like this;
“DecimalFormat df = new DecimalFormat(“###.###);
System.out.println(df.format(PI));”
Using this allows you to set up behavior for rounding, which actually gives you more control over the output than using something else like the string format we showed you above. It is useful for many things.
However, when it comes to rounding, you want to use BigDecimal, which we will look at now.
Using BigDecimal
What if you have doubles, and you need to round them in Java? Well, this is where BigDecimal comes in really handy.
In order to round your doubles to an n decimal place, you want to write up a helped method. If you are unfamiliar with this, we will give you an example.
“Private static double round(double value, int places) {
If (places < 0) throw new IllegalArgumentException ( );
Big Decimal bd = New BigDecimal (Double.toString(value));
Bd = bd.setScales(places, Roundingmode.HALF_UP);
Return bd.doubleValue ( );
} “
However, there is something that you need to notice in using this. When you are using BigDecimal for this purpose, you always have to use the BigDecimal(String) constructor.
Why?
Well, it stops you from having any problems with your inexact values becoming misrepresented.
You could, however, also get the same result if you use the Apache Commons Math library instead
If you were to use this, the code would look somewhat similar, and it could render the exact same results, however, for some it may be easier to use this instead.
Here is an example of the code you should expect from this.
“<dependency.
<groupId.org.apache.commons,/groupId>
<artifactId>commons-math3</artifactId>
<version>3.5</version>
</dependency>”
Once you have added this library to your project, you can then use the Precision.round() method. This method will take only two arguments, which will be scale and value.
However, by default, it pretty much uses the exact same HALF-UP method for rounding as a helper method would, so it is going to deliver results that are pretty much exactly the same.
Note that you could change any rounding behavior by simply passing the desired method of rounding as a sort of third parameter.
It is not too difficult to use, however for some using this may be a bit easier than alternatives.
To Conclude
There are plenty of methods for rounding in Java. However, rounding is fairly easy to do, you just want to make sure that you employ the right methods and understand the code and how it will work in your favor.
Always be mindful of values and the type of numbers you are dealing with before you start rounding.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.